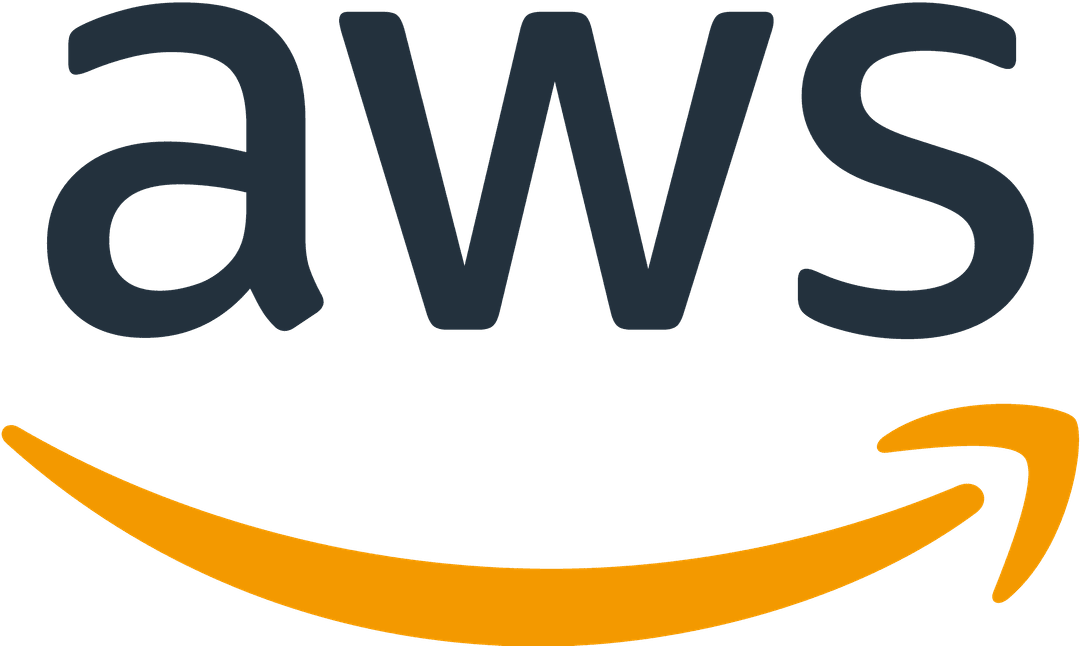
aws-sdk-client-mockの使い方
2024-01-22
node.jsawsaws-sdk-client-mock設定
パッケージインストール
1$ npm -i aws-sdk-client-mock aws-sdk-client-mock-jest
jest.config.js
1/* 2 * For a detailed explanation regarding each configuration property, visit: 3 * https://jestjs.io/docs/configuration 4 */ 5 6module.exports = { 7 clearMocks: true, 8 collectCoverage: true, 9 coverageDirectory: "coverage", 10 coveragePathIgnorePatterns: [ 11 "/node_modules/" 12 ], 13 testEnvironment: 'node', 14 moduleFileExtensions: ["js","mjs","json","node",], 15 moduleNameMapper: { 16 "\\.(jpg|jpeg|png|gif|eot|otf|webp|svg|ttf|woff|woff2|mp4|webm|wav|mp3|m4a|aac|oga)$": 17 "<rootDir>/__mocks__/fileMock.js", 18 "\\.(css|less)$": "<rootDir>/__mocks__/styleMock.js", 19 }, 20 testMatch: [ 21 "**/__tests__/**/*.[jt]s?(x)", 22 "**/?(*.)+(spec|test).[tj]s?(x)" 23 ], 24 testPathIgnorePatterns: [ 25 "/node_modules/" 26 ], 27 transform: { 28 '^.+\\.m?js?$': 'babel-jest', 29 }, 30};
DynamoDBからItem取得するコード
1import { DynamoDBDocumentClient, GetCommand } from "@aws-sdk/lib-dynamodb"; 2import { DynamoDBClient } from '@aws-sdk/client-dynamodb'; 3 4export const initDynamoClient = () => { 5 const marshallOptions = { 6 removeUndefinedValues: true, 7 }; 8 const translateConfig = { marshallOptions }; 9 const DynamoDBclient = new DynamoDBClient({ 10 region: 'ap-northeast-1' 11 }); 12 13 const dynamo = DynamoDBDocumentClient.from( DynamoDBclient, translateConfig ); 14 return dynamo; 15} 16 17export const response = (statusCode, body) => { 18 return { 19 statusCode: statusCode, 20 headers: { 21 "Access-Control-Allow-Headers" : "Content-Type", 22 "Access-Control-Allow-Origin": "*", 23 "Access-Control-Allow-Methods": "GET" 24 }, 25 body: JSON.stringify( 26 body 27 ), 28 }; 29}; 30 31export const handler = async (event) => { 32 try { 33 const dynamo = initDynamoClient(); 34 const id = event.pathParameters.id; 35 const dynamo_data = await dynamo.send( 36 new GetCommand({ 37 TableName: "Dynamo_test", 38 Key: { 39 id: id, 40 }, 41 }) 42 ); 43 if (dynamo_data.Item === undefined) { 44 return response(404, {"message": "not found."}); 45 } else { 46 return response(200, dynamo_data.Item); 47 } 48 } catch (error) { 49 console.log(error); 50 return { 51 statusCode: 500, 52 headers: { 53 "Access-Control-Allow-Headers" : "Content-Type", 54 "Access-Control-Allow-Origin": "*", 55 "Access-Control-Allow-Methods": "GET" 56 }, 57 body: JSON.stringify({"message": "500err"}), 58 }; 59 } 60};
testコード
1import { handler as getItem } from "../get_dynamo.mjs"; 2import { DynamoDBDocumentClient, GetCommand } from "@aws-sdk/lib-dynamodb"; 3import { mockClient } from "aws-sdk-client-mock"; 4 5const ddbMock = mockClient(DynamoDBDocumentClient) 6 7describe("handler get item", () => { 8 beforeEach(() => { 9 ddbMock.reset() 10 }) 11 12 it("get item test", async () => { 13 const event = { 14 "pathParameters": { 15 "id": "test" 16 } 17 }; 18 const expectValue = { 19 Item: { id: "test", value: "memo" } 20 }; 21 ddbMock.on(GetCommand).resolves(expectValue) 22 const result = await getItem(event) 23 expect(result).toEqual({ 24 statusCode: 200, 25 headers: { 26 "Access-Control-Allow-Headers" : "Content-Type", 27 "Access-Control-Allow-Origin": "*", 28 "Access-Control-Allow-Methods": "GET" 29 }, 30 body: JSON.stringify( 31 {id:"test",value:"memo"} 32 ) 33 }) 34 }) 35 36 it("item not found test", async () => { 37 const event = { 38 "pathParameters": { 39 "id": "notfoundID" 40 } 41 }; 42 const expectValue = { 43 Item: undefined 44 }; 45 ddbMock.on(GetCommand).resolves(expectValue) 46 const result = await getItem(event) 47 expect(result).toEqual({ 48 statusCode: 404, 49 headers: { 50 "Access-Control-Allow-Headers" : "Content-Type", 51 "Access-Control-Allow-Origin": "*", 52 "Access-Control-Allow-Methods": "GET" 53 }, 54 body: JSON.stringify( 55 {"message": "not found."} 56 ), 57 }); 58 }) 59 60 it("handler response test", async () => { 61 const event = { 62 "pathParameters": { 63 "id": "test" 64 } 65 }; 66 const expectValue = { 67 Item: { id: event.pathParameters.id, value: "memo" } 68 }; 69 ddbMock.on(GetCommand).resolves(expectValue) 70 const result = await getItem(event) 71 expect(result).toEqual({ 72 statusCode: 200, 73 headers: { 74 "Access-Control-Allow-Headers" : "Content-Type", 75 "Access-Control-Allow-Origin": "*", 76 "Access-Control-Allow-Methods": "GET" 77 }, 78 body: JSON.stringify( 79 expectValue.Item 80 ), 81 }); 82 }) 83}) 84 85describe("handler get item error test", () => { 86 beforeEach(() => { 87 ddbMock.reset() 88 }) 89 90 it("handler error test", async () => { 91 const event = { 92 "pathParameters": { 93 "id": "test" 94 } 95 }; 96 const expectValue = "ERR" 97 ddbMock.on(GetCommand).rejects(expectValue) 98 const result = await getItem(event) 99 expect(result).toEqual({ 100 statusCode: 500, 101 headers: { 102 "Access-Control-Allow-Headers" : "Content-Type", 103 "Access-Control-Allow-Origin": "*", 104 "Access-Control-Allow-Methods": "GET" 105 }, 106 body: JSON.stringify( 107 {"message": "500err"} 108 ), 109 }); 110 }) 111})